Xamarin.Android と MvvmCross 4 でリスト形式の AlertDialog を表示します。
リスト形式の AlertDialog
普通のリスト形式の場合。MvvmCross は関係ありません。
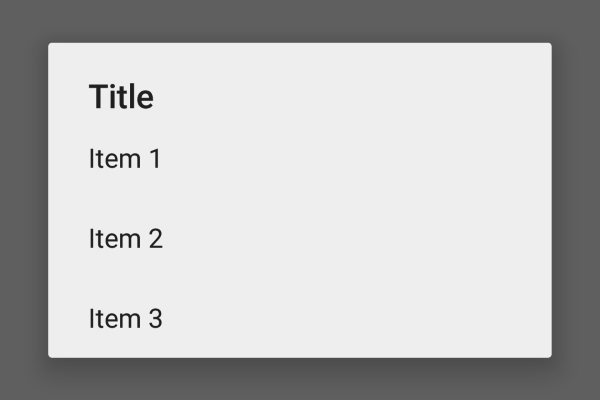
var alert = new AlertDialog.Builder(this);
alert.SetTitle("Title");
var items = new string[]
{
"Item 1",
"Item 2",
"Item 3",
};
alert.SetItems(items, (s, a) =>
{
var selectedItem = items[a.Which];
});
alert.Show();
アイコン付きリスト形式の AlertDialog
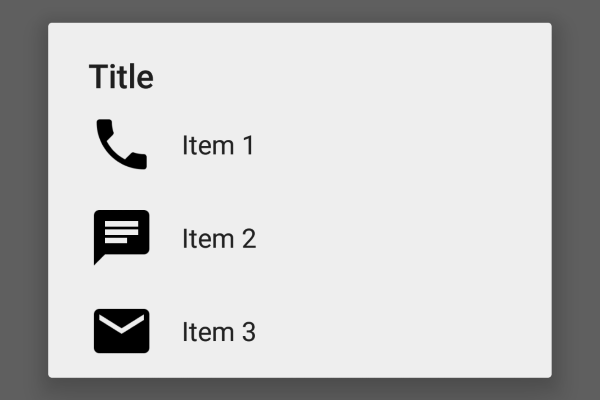
AlertDialog の表示
呼び出し部分から。
var alert = new AlertDialog.Builder(this);
alert.SetTitle("Title");
var items = new List<ListItem>()
{
new ListItem("Item 1", "res:ic_call_black"),
new ListItem("Item 2", "res:ic_chat_black"),
new ListItem("Item 3", "res:ic_email_black"),
};
var adapter = new ListAdapter(this, (IMvxAndroidBindingContext)BindingContext);
adapter.ItemsSource = items;
alert.SetAdapter(adapter, (s, a) =>
{
var selectedItem = items[a.Which];
});
alert.Show();
MvxImageView の ImageUrl にリソース画像を指定するには「res:」を付けます。
リスト項目の View
アイコンとテキストを表示する MvxImageView と TextView を配置した axml ファイルを用意します。ImageUrl と Text プロパティを Binding 指定しています。
xml version="1.0" encoding="utf-8"
<LinearLayout xmlnsandroid="http://schemas.android.com/apk/res/android"
xmlnslocal="http://schemas.android.com/apk/res-auto"
androidorientation="horizontal"
androidlayout_width="match_parent"
androidlayout_height="56dp">
<MvxImageView
androidlayout_marginTop="8dp"
androidlayout_marginBottom="8dp"
androidlayout_marginLeft="24dp"
androidlayout_width="40dp"
androidlayout_height="40dp"
localMvxBind="ImageUrl ImageUrl" />
<TextView
androidlayout_marginTop="8dp"
androidlayout_marginBottom="8dp"
androidlayout_marginLeft="16dp"
androidlayout_marginRight="24dp"
androidgravity="center_vertical"
androidlayout_width="0dp"
androidlayout_height="match_parent"
androidlayout_weight="1"
androidellipsize="end"
androidtext="example"
localMvxBind="Text Text"
androidtextColor="@color/primary_text"
androidtextSize="16sp" />
</LinearLayout>
リスト項目の Class
リストの項目を表すクラスを作ります。
private class ListItem
{
public string Text { get; set; }
public string ImageUrl { get; set; }
public ListItem(string text, string imageUrl)
{
this.Text = text;
this.ImageUrl = imageUrl;
}
}
カスタム Adapter
カスタム Adapter を作り、GetView 内で作成した View の Resource ID を指定します。
private class ListAdapter : MvxAdapter
{
public ListAdapter(Context context, IMvxAndroidBindingContext bindingContext) : base(context, bindingContext)
{
}
protected override View GetView(int position, View convertView, ViewGroup parent, int templateId)
{
templateId = Resource.Layout.ListItem;
return base.GetView(position, convertView, parent, templateId);
}
}